Architecture
Single-node (in-memory)
complete
SurrealDB can be configured to run in a single-node
memory-only runtime, enabling multi-reader,
single-writer querying and data analysis. This is
suitable for testing and for data which fits inside
the memory capacity of a single machine.
Single-node (SurrealKV)
beta
Our own native storage engine, written in Rust
supports multiple concurrent writers and readers.
SurrealKV introduces versioned queries over time,
enabling immutable data querying, data change
auditing, historic aggregate query analysis, and
versioned queries on the graph.
Distributed (SurrealKV)
in development
For highly-available setups, SurrealKV will support
the ability to horizontally scale to multiple
terabytes of data. As a distributed cluster
SurrealKV will enable versioned queries over time,
enabling immutable data querying, data change
auditing, historic aggregate query analysis, and
versioned queries on the graph.
When wanting persistent data storage on a
single-node, on-disk storage can be used to enable
larger data storage capabilities. RocksDB is optimised for high performance and fast storage
on SSDs.
Platform
Multi-tenancy data separation
complete
Split data into namespaces and databases. There is
no limit to the number of databases under each
namespace, with the ability to switch between
databases inside queries and transactions.
Schemafull or schemaless
complete
Store unstructured nested data with any columns, or
limit data stored to only specific columns or
fields. Get started quickly without having to define
every column, and move to schemafull when your data
model is defined.
Multi-table, multi-row transactions
complete
As a fully ACID compliant database, SurrealDB allows
you to run transactions across multiple-rows, and
across multiple different tables. There is no limit
to the length of time a transaction can run.
Versioned temporal tables
in development
Versioned temporal tables enable the option to 'go
back in time' when querying your data. See how data
looked before changes were made, or restore to a
particular point-in-time.
Table fields
complete
When a table is defined as schemafull, only data
allowed by defined fields will be stored. Table
fields can be nested, and can be limited to a
certain data type.
VALUE
clauses can be
used to ensure a default value is always specified if
no data is entered.
Table events
complete
Table events can be triggered after any change or
modification to the data in a record. Each trigger
is able to see the
$before
and $after
value of the record, enabling advanced custom logic
with each trigger.
Table indexes
complete
Table indexes improve data querying performance, and
also allow for
UNIQUE
values in a table.
Table indexes can be specified for a column, multiple
columns, and have support for all nested fields including
arrays and objects.
-- Specify a field on the user table DEFINE FIELD email ON TABLE user TYPE string ASSERT string::is::email($value); -- Add a unique index on the email field to prevent duplicate values DEFINE INDEX email ON TABLE user COLUMNS email UNIQUE; -- Create a new event whenever a user changes their email address DEFINE EVENT email ON TABLE user WHEN $before.email != $after.email THEN ( CREATE event SET user = $this, time = time::now(), value = $after.email, action = 'email_changed' );
Table constraints
complete
Each defined table field supports an
ASSERT
clause which acts as a constraint on the data. This
clause enables advanced SurrealQL statements which can
ensure that the $value
entered is within
certain parameters. Each clause is also able to see the
$before
and $after
value of
the record, enabling advanced custom logic with each
trigger.
-- Specify a field on the user table DEFINE FIELD countrycode ON user TYPE string -- Ensure country code is ISO-3166 ASSERT $value = /[A-Z]{3}/ -- Set a default value if empty VALUE $value OR $before OR 'GBR' ;
Full text indexing and filtering
complete
The ability to define full-text indexes, with
functionality to search through the full-text
index on a table. Searches support field
queries, configurable text analyzers, relevance
matching, highlighting with offsets extraction.
-- Define a text analyzer DEFINE ANALYZER en TOKENIZERS camel,class FILTERS snowball(English); -- Define a search index for a field on the book table DEFINE INDEX search_title ON book COLUMNS title SEARCH ANALYZER en BM25 HIGHLIGHTS; -- Select all books who match given keywords SELECT search::score(1) AS score, search::highlight('<b>', '</b>', 1) AS title FROM book WHERE title @1@ 'rust web' ORDER BY score DESC;
Vector embedding indexing
complete
Indexing of vector embeddings, with support for
euclidean
distance metrics. Vector embeddings can be used
for similarity matching, and for advanced data analysis.
-- Add vector embedding data to record content CREATE article:1 SET embedding = [1, 2, 3, 4]; CREATE article:2 SET embedding = [4, 5, 6, 7]; CREATE article:3 SET embedding = [8, 9, 10, 11]; -- Define a vector embedding index for a field on the article table DEFINE INDEX mt_obj ON vec FIELDS embedding MTREE DIMENSION 4 DIST EUCLIDEAN;
Aggregate analytics views
complete
Aggregate views let you pre-compute analytics
queries as data is written to SurrealDB.
Similarly to an index, a table view lets you
select, aggregate, group, and order data, with
support for moving averages, time-based
windowing, and attribute-based counting.
Pre-defined aggregate views are efficient and
performant, with only a single record
modification being made for every write.
-- Drop all writes to the reading table. We don't need every reading. DEFINE TABLE reading DROP; -- Define a table as a view which aggregates data from the reading table DEFINE TABLE temperatures_by_month AS SELECT count() AS total, time::month(recorded_at) AS month, math::mean(temperature) AS average_temp FROM reading GROUP BY city ; -- Add a new temperature reading with some basic attributes CREATE reading SET temperature = 27.4, recorded_at = time::now(), city = 'London', location = (-0.118092, 51.509865) ;
Live queries and record changes
complete
Live SQL queries allow for advanced filtering of the
changes to specific documents, documents which match
a particular filter, or all documents in a specific
table. Live SQL queries can send the fully-updated
document, or only the document changesets, by using
the efficient DIFF-MATCH-PATCH algorithm for
highly-performant web-based data syncing.
-- Subscribe to all matching document changes LIVE SELECT * FROM document WHERE account = $auth.account OR public = true ; -- Subscribe to all changes to a single record LIVE SELECT * FROM post:c569rth77ad48tc6s3ig; -- Stop receiving change notifications KILL "1986cc4e-340a-467d-9290-de81583267a2";
Global parameters
complete
Global parameters can be used to store values
across the database, which are then accessible
to all queries.
-- Define a global parameter which will be accessible to all queries. DEFINE PARAM $STRIPE VALUE "https://api.stripe.com/payments/new"; -- Use the defined global parameter in all queries on the database. DEFINE EVENT payment ON TABLE order WHEN $event = 'CREATE' THEN http::post($STRIPE, $value);
Data model
Basic types
complete
Support for booleans, strings, and numerics is built
in by default. Numeric values default to
decimal
based numbers, but can be stored as int
or float
values for 64 bit integer or
64 bit floating point precision.
Empty values
complete
Values can be
NONE
, or NULL
. A field which is NONE
does not have any
data stored, while NULL
values are values
which are entered but empty.
Arrays
complete
SurrealDB has native support for arrays, with no
limit to the depth of nesting within arrays. Arrays
can contain any other data value.
Objects
complete
Embedded object types are an integral feature of
SurrealDB, with no limit to the depth of nesting for
objects.
Durations
complete
Any duration from nanoseconds to weeks can be stored
and used for calculations. Durations can be added to
datetimes, and to other durations.
Datetimes
complete
Support for dates and datetimes in ISO-8601 format
are supported. All dates are converted and stored in
the UTC timezone.
Geometries
complete
SurrealDB makes working with GeoJSON easy, with
support for
Point
, Line
, Polygon
, MultiPoint
, MultiLine
, MultiPolygon
, and Collection
values. SurrealQL automatically
detects GeoJSON objects converting them into a single
data type.
UPDATE city:london SET centre = (-0.118092, 51.509865), boundary = { type: "Polygon", coordinates: [[ [-0.38314819, 51.37692386], [0.1785278, 51.37692386], [0.1785278, 51.61460570], [-0.38314819, 51.61460570], [-0.38314819, 51.37692386] ]] } ;
Futures
complete
Values which should be computed only when
outputting data, can be stored as futures. These
values are stored in SurrealDB as SurrealQL
code, and are calculated only when output as
part of a
SELECT
clause.
UPDATE product SET name = "SurrealDB", launch_at = <datetime> "2021-11-01", countdown = <future> { launch_at - time::now() } ;
Casting
complete
In SurrealDB, all data values are strongly
typed. Values can be cast and converted to other
types using specific casting operators. These
include
bool
, int
, float
, string
, number
, decimal
, datetime
, and duration
casts.
UPDATE person SET waist = <int> "34", height = <float> 201, score = <decimal> 0.3 + 0.3 + 0.3 + 0.1 ;
Strict typing
complete
With a strict typing system, SurrealQL ensures
that document structures are easier to
understand, and any data conforms to the defined
record schema. Advanced types for arrays and
record links, ensure that related data works in
the same way as basic types.
// Ensure that a record field must be a number. DEFINE FIELD age ON person TYPE number; // Allow the field to be optional or a number. DEFINE FIELD age ON person TYPE option<number>; // Ensure that a record link is specified and of a specific type. DEFINE FIELD author ON book TYPE record<person>; // Allow a field to be optional and of a selection of types. DEFINE FIELD pet ON user TYPE option<record<cat | dog>>; // Allow a field to be one of multiple types. DEFINE FIELD rating ON film TYPE float | decimal; // Ensure that a field is an a array of unique values of a certain length. DEFINE FIELD tags ON person TYPE set<string, 5>;
SurrealQL
SELECT, CREATE, UPDATE, DELETE statements
complete
Manipulation and querying of data in SurrealQL is
done using the
SELECT
, CREATE
, UPDATE
, and DELETE
methods.
These enable selecting or modifying individual records,
or whole tables. Each statement supports multiple different
tables or record types at once.
-- Create a new article record with a specific id CREATE article:surreal SET name = "SurrealDB: The next generation database"; -- Update the article record, and add a new field UPDATE article:surreal SET time.created = time::now(); -- Select all matching articles SELECT * FROM article, post WHERE name CONTAINS 'SurrealDB'; -- Delete the article DELETE article:surreal;
RELATE statements
complete
The
RELATE
statement adds graph edges
between records in SurrealDB. It follows the convention
of vertex -> edge -> vertex
or noun -> verb -> noun
, enabling the addition of metadata to the edge
record.
-- Add a graph edge between user:tobie and article:surreal RELATE user:tobie->write->article:surreal SET time.written = time::now() ; -- Add a graph edge between specific users and developers LET $from = (SELECT users FROM company:surrealdb); LET $devs = (SELECT * FROM user WHERE tags CONTAINS 'developer'); RELATE $from->like->$devs UNIQUE SET time.connected = time::now() ;
INSERT statements
complete
The
INSERT
statement resembles the traditional
SQL statement, enabling users to get started quickly.
It supports the creation of records using a VALUES
clause, or by specifying the record data as an
object.
INSERT INTO company { name: 'SurrealDB', founded: "2021-09-10", founders: [person:tobie, person:jaime], tags: ['big data', 'database'] }; INSERT IGNORE INTO company (name, founded) VALUES ('SurrealDB', '2021-09-10') ON DUPLICATE KEY UPDATE tags += 'developer tools' ;
FOR statements
completeFOR
statements enable simplified iteration
over data, or for advanced logic when dealing with
nested arrays or recursive functions, within code
blocks or custom functions.
FOR $person IN (SELECT VALUE id FROM person) { CREATE gift CONTENT { recipient: $person, type: "ticket", date: time::now(), event: "SurrealDB World", }; };
THROW statements
complete
The
THROW
statement statement can be
used to return custom error types, which allow for
building advanced programming and business logic
right within the database and authentication engine.
FOR $user IN $input { IF $user.age < 18 { THROW "Only adults should be inserted"; } IF $user.country != 'USA' { THROW $user.country + " is not a valid country"; } };
Parameters
complete
Parameters can be used to store values or result
sets, and can be used as stored parameters in client
code.
Subqueries
complete
Recursive subqueries are useful for advanced
querying or modification of values, whilst
simplifying the overall query.
Nested field queries
complete
In SurrealQL any nested array or object value can be
accessed and manipulated using traditional dot
notation
.
, or array notation []
.
Maths operators
complete
Maths operators can be used to perform complex
mathematical calculations directly in SurrealQL.
Geo operators
complete
Geospatial operators enable geospatial
containment and intersection operators on
geospatial types.
Set operators
complete
Advanced set operators can be used to detect
whether one or multiple values are included
within an array. Fuzzy matching and regex
matching can also be used for advanced
filtering.
-- Use mathematical operators to calculate value SELECT * FROM temperature WHERE (celsius * 1.8) + 32 > 86.0; -- Use geospatial operator to detect polygon containment SELECT * FROM restaurant WHERE location INSIDE { type: "Polygon", coordinates: [[ [-0.38314819, 51.37692386], [0.1785278, 51.37692386], [0.1785278, 51.61460570], [-0.38314819, 51.61460570], [-0.38314819, 51.37692386] ]] }; -- Select all people whose tags contain "tag1" OR "tag2" SELECT * FROM person WHERE tags CONTAINSANY ["tag1", "tag2"]; -- Select all people who have any email address ending in 'gmail.com' SELECT * FROM person WHERE emails.*.value ?= /gmail.com$/;
Maths constants
complete
SurrealQL has a number of built-in constants for
advanced mathematical expressions and calculations,
including
math::E
, math::FRAC_1_PI
, math::FRAC_1_SQRT_2
, math::FRAC_2_PI
, math::FRAC_2_SQRT_PI
, math::FRAC_PI_2
, math::FRAC_PI_3
, math::FRAC_PI_4
, math::FRAC_PI_6
, math::FRAC_PI_8
, math::LN_10
, math::LN_2
, math::LOG10_2
, math::LOG10_E
, math::LOG2_10
, math::LOG2_E
, math::PI
, math::SQRT_2
,
and math::TAU
.
Expressions
complete
SurrealQL supports fetching data using dot
notation
.
, array notation []
, and graph semantics ->
. SurrealQL
enables records to link to other records and
traverses all embedded links or graph
connections as desired. When traversing and
fetching remote records SurrealQL enables
advanced filtering using traditional WHERE
clauses.
-- Select a nested array, and filter based on an attribute SELECT emails[WHERE active = true] FROM person; -- Select all 1st, 2nd, and 3rd level people who this specific person record knows, or likes, as separate outputs SELECT ->knows->(? AS f1)->knows->(? AS f2)->(knows, likes WHERE influencer = true AS e3)->(? AS f3) FROM person:tobie; -- Select all person records (and their recipients), who have sent more than 5 emails SELECT *, ->sent->email->to->person FROM person WHERE count(->sent->email) > 5; -- Select other products purchased by people who purchased this laptop SELECT <-purchased<-person->purchased->product FROM product:laptop; -- Select products purchased by people in the last 3 weeks who have purchased the same products that we purchased SELECT ->purchased->product<-purchased<-person->(purchased WHERE created_at > time::now() - 3w)->product FROM person:tobie;
Complex Record IDs
complete
SurrealDB supports the ability to define record
IDs using arrays and objects. These values sort
correctly, and can be used to store values or
recordings in a timeseries context.
// Set a new parameter LET $now = time::now(); // Create a record with a complex ID using an array CREATE temperature:['London', $now] SET location = 'London', date = $now, temperature = 23.7 ; // Create a record with a complex ID using an object CREATE temperature:{ location: 'London', date: $now } SET location = 'London', date = $now, temperature = 23.7 ;
Record ID ranges
complete
SurrealDB supports the ability to query a range
of records, using the record ID. The record ID
ranges, retrieve records using the natural
sorting order of the record IDs. These range
queries can be used to query a range of records
in a timeseries context.
-- Select all person records with IDs between the given range SELECT * FROM person:1..1000; -- Select all records for a particular location, inclusive SELECT * FROM temperature:['London', NONE]..=['London', time::now()]; -- Select all temperature records with IDs less than a maximum value SELECT * FROM temperature:..['London', '2022-08-29T08:09:31']; -- Select all temperature records with IDs greater than a minimum value SELECT * FROM temperature:['London', '2022-08-29T08:03:39']..; -- Select all temperature records with IDs between the specified range SELECT * FROM temperature:['London', '2022-08-29T08:03:39']..['London', '2022-08-29T08:09:31'];
SurrealML
Custom machine learning models
complete
Use SurrealML to train custom machine learning
models in Python, using PyTorch, Tensorflow, or
Sklearn. The models are stored in a custom
.surml
data-format, enabling the
model to be run consistently and safely in
Python, Rust, or SurrealDB. Models can then be
imorted into SurrealDB, allowing for Inference
directly against any data in the database.
from surrealml import SurMlFile # Specify the trained model, model name, and model inputs file = SurMlFile(model=model, name="house::price::prediction", inputs=test_inputs) # Add a description for this model that can be viewed in teh database file.add_description("This model predicts the price of a house based on its square footage and number of floors.") # Add a version identifier which enables specific model versions to be run separately file.add_version("0.3.0") # Optionally define named input fields and data normalisation functions file.add_column("squarefoot") file.add_column("num_floors") file.add_normaliser("squarefoot", "z_score", squarefoot_mean, squarefoot_std) file.add_normaliser("num_floors", "z_score", num_floors_mean, num_floors_std) # Optionally specify named output fields with normalisation functions file.add_output("house_price", "z_score", house_price_mean, house_price_std) # Save the .surml file, ready to execute or import into the database file.save("./my_model.surml")
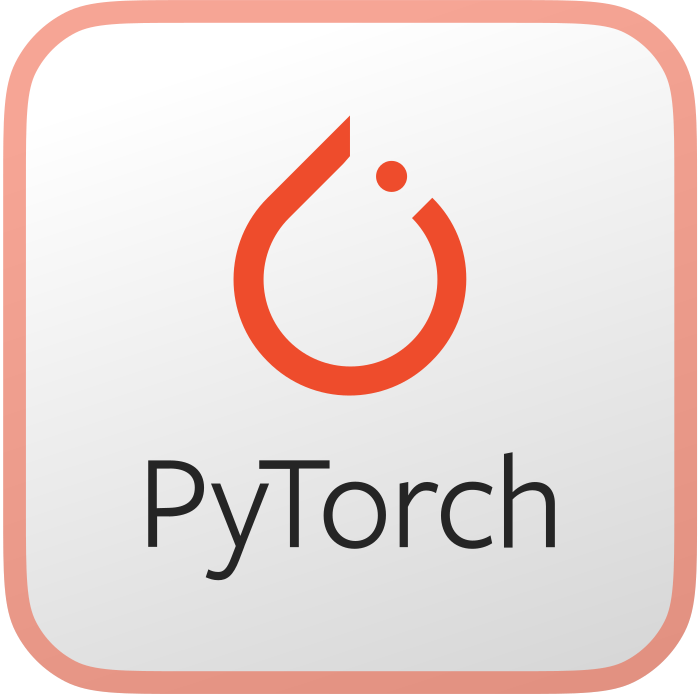
PyTorch
available
PyTorch models are supported natively with
SurrealML when running in Python, or within
SurrealDB.
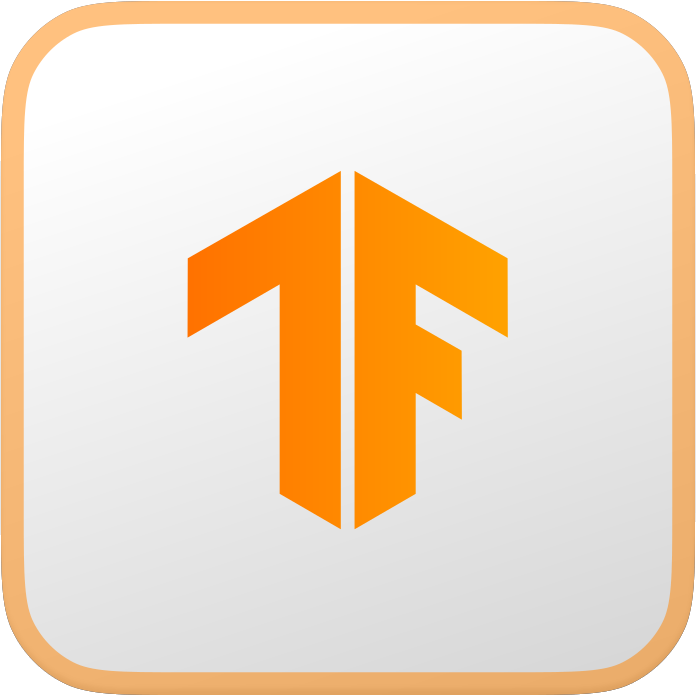
Tensorflow
available
Tensorflow models are supported natively with
SurrealML when running in Python, or within
SurrealDB.
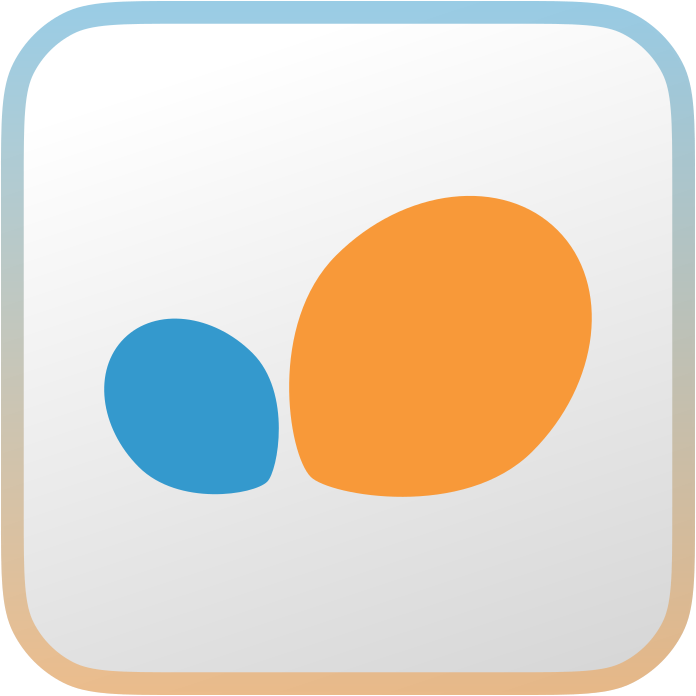
Sklearn
available
Sklearn models are supported natively with
SurrealML when running in Python, or within
SurrealDB.
Import models into SurrealDB
complete
SurrealDB allows developers the choice of storing SurrealML models on local storage, or remote storage including Amazon S3, Google Cloud Storage, or Azure Storage.
Export models from SurrealDB
complete
SurrealDB allows developers to store multiple versions of each SurrealML model, and to export each model from the database as a binary file.
Model inference in Python
complete
Inference on
.surml
model files in Python
allows for consistent and reproducible modeel
computation in development, continuous integration,
testing, or production environments.
from surrealml import SurMlFile # Load the model file from disk model = SurMlFile.load("./my_model.surml") # Perform raw vector compute against the model output = model.raw_compute([1.0, 2.0], [1, 2]) # Perform named buffered compute against the model output = model.buffered_compute({ "squarefoot": 3200.0, "num_floors": 2.0 })
Model inference in SurrealDB
complete
Model inference within SurrealDB is powered by a
Rust-native runtime, backed by ONNX, with support
for PyTorch, Tensorflow, and Sklearn models. This
secure, and performant runtime allows for CPU and
GPU model inference right alongside the data within
the database. Field are automatically mapped to the
right position for the model input tensors, and
normalisations, if specified, are automatically
applied to any input values, reducing errors of
implementation of the models when deployed.
-- Perform raw computation against the imported model RETURN ml::house::price::prediction<0.3.0>( [1.0, 2.0], [1, 2] ); -- Perform named buffered computation against the imported model SELECT *, ml::house::price::prediction<0.3.0>({ squarefoot: squarefoot_col, num_floors: num_floors_col }) AS price_prediction FROM property_listing WHERE price_prediction > 177206.21875 ;
Functions
Array functions
complete
Functions for manipulation, joining, and diffing of
arrays are built into SurrealDB as standard.
Http functions
complete
HTTP functions can be used for remote trigger events
and webhook functionality.
Math functions
complete
Math functions can be used for complex statistical
analysis of numbers and sets of numbers.
Parsing functions
complete
Parsing functions can be used for parsing and
extracting individual parts or urls, emails, and
domains.
Rand functions
complete
Random generation functions can be used to generate
random values, numbers, strings, UUIDs, and
datetimes.
Search functions
complete
Functions related to the full-text search
capabilities, such as calculating relevance scores
or highlighting content.
String functions
complete
Functions for string manipulation enable
modification and processing of strings.
Type functions
complete
Type checking functions can be used to check the
type of a value, which is useful in custom function
definitions.
Vector functions
complete
A collection of essential vector operations that
provide foundational functionality for numerical
computation, machine learning, and data analysis.
Geo functions
complete
Geospatial functions can be used for converting
between geohash values, and for calculating the
distance, bearing, and area of GeoJSON data
types.
SELECT * FROM geo::hash::encode( (-0.118092, 51.509865) );
Time functions
complete
Time functions can be used to manipulate dates
and times - with support for rounding, and
extracting specific parts of datetimes.
SELECT time::floor(created_at, 1w) FROM user;
Count functions
complete
SurrealDB supports general count functionality
for counting total values, or for aggregate
grouping. It's also possible to count only those
expressions which result in a truthy value.
SELECT count(age > 18) FROM user GROUP ALL;
Validation functions
complete
Validation functions can be used to determine
that field values match a certain pattern
including hexadecimal, alphanumeric, ascii,
numeric, or email addresses.
SELECT email, string::is::email(email) AS valid FROM user;
Embedded JavaScript functions
complete
JavaScript functions can be used for more
complex functions and triggers. Each JavaScript
function iteration runs with its own context
isolation - with the current record data passed
in as the execution context or
this
value.
CREATE film SET ratings = [ { rating: 6, user: user:bt8e39uh1ouhfm8ko8s0 }, { rating: 8, user: user:bsilfhu88j04rgs0ga70 }, ], featured = function() { return this.ratings.filter(r => { return r.rating >= 7; }).map(r => { return { ...r, rating: r.rating * 10 }; }); } ;
Custom functions
complete
Custom functions allow for complicated or
repeated user-defined code, to be run seamlessly
within any query across the database. Custom
functions support typed arguments, and multiple
nested queries with custom logic.
-- Define a global function which can be used in any query DEFINE FUNCTION fn::get::person($first: string, $last: string, $birthday: string) { LET $person = SELECT * FROM person WHERE [first, last, birthday] = [$first, $last, $birthday]; RETURN IF $person[0].id THEN $person[0] ELSE CREATE person SET first = $first, last = $last, birthday = $birthday END; }; -- Call the global custom function, receiving the returned result LET $person = fn::get::person('Tobie', 'Morgan Hitchcock', '2022-09-21');
Permissions
Root access
complete
Root access enables full data access for all data in
SurrealDB. Root access can be limited to specific
IPv4
or IPv6
IP addresses.
Namespace access
complete
Namespace access enables full data access for all
databases under a specific namespace. This access
level is controlled using custom defined usernames
and passwords.
Database access
complete
Database access enables full data access to a
specific database under a specific namespace. This
access level is controlled using custom defined
usernames and passwords.
Scope access
complete
Scope access is the powerful functionality which
enables SurrealDB to operate as a web database.
Flexible authentication and access rules enable
fine-grained access to tables and fields with the
highest security, whilst ensuring that performance
is affected as little as possible.
3rd party authentication
complete
If authentication with a 3rd party OAuth provider is
desired, specific tokens can be used for
authentication with SurrealDB.
ES256
, ES384
, ES512
, HS256
, HS384
, HS512
, PS256
, PS384
, PS512
, RS256
, RS384
, and RS512
algorithms are supported.
-- Enable scope authentication directly in SurrealDB DEFINE SCOPE account SESSION 24h SIGNUP ( CREATE user SET email = $email, pass = crypto::argon2::generate($pass) ) SIGNIN ( SELECT * FROM user WHERE email = $email AND crypto::argon2::compare(pass, $pass) ) ;
// Signin and retrieve a JSON Web Token let jwt = fetch('https://api.surrealdb.com/signup', { method: 'POST', headers: { Accept: 'application/json', NS: 'google', // Specify the namespace DB: 'gmail', // Specify the database }, body: JSON.stringify({ NS: 'google', DB: 'gmail', SC: 'account', email: 'tobie@surrealdb.com', pass: 'a85b19*1@jnta0$b&!', }), });
Table permissions
complete
Fine-grained table permissions can be used to
prevent users from accessing data which they
shouldn't see. Independent permissions for
selecting, creating, updating, and deleting data are
supported, ensuring fine-grained control over all
data in SurrealDB.
-- Specify access permissions for the 'post' table DEFINE TABLE post SCHEMALESS PERMISSIONS FOR select -- Published posts can be selected WHERE published = true -- A user can select all their own posts OR user = $auth.id FOR create, update -- A user can create or update their own posts WHERE user = $auth.id FOR delete -- A user can delete their own posts WHERE user = $auth.id -- Or an admin can delete any posts OR $auth.admin = true ;
Connectivity
REST API
complete
All tables and data can be queried using a
traditional Key-Value REST API. In addition,
SurrealQL statements can be submitted to the REST
API for custom query logic.
## Execute a SurrealQL query localhost % curl -X POST https://api.surrealdb.com/sql ## Interact with a table localhost % curl -X GET https://api.surrealdb.com/key/user localhost % curl -X POST https://api.surrealdb.com/key/user localhost % curl -X DELETE https://api.surrealdb.com/key/user ## Interact with a record localhost % curl -X GET https://api.surrealdb.com/key/user/tobie localhost % curl -X PUT https://api.surrealdb.com/key/user/tobie localhost % curl -X POST https://api.surrealdb.com/key/user/tobie localhost % curl -X PATCH https://api.surrealdb.com/key/user/tobie localhost % curl -X DELETE https://api.surrealdb.com/key/user/tobie
SurrealQL over HTTP/WS
complete
SurrealQL querying and data modification is
supported over WebSockets for bi-directional
communication, and real-time updates.
CBOR RPC over HTTP/WS
complete
Querying and data modification is available using
JSON-RPC over WebSockets, enabling easier
implementation of 3rd party libraries.
JSON RPC over HTTP/WS
complete
SurrealQL querying and data modification is
supported over WebSockets for bi-directional
communication, and real-time updates.
Binary RPC over HTTP/WS
complete
Querying and data modification is available using
JSON-RPC over WebSockets, enabling easier
implementation of 3rd party libraries.
GraphQL schema generation
experimental
Support for automatic generation of GraphQL schema,
from database tables, fields, types, and custom
functions.
GraphQL querying
experimental
Support for querying all data using GraphQL, with
embedded and remote record fetching.
GraphQL mutations
planned 2.x
Support for modifying and updating any data using
GraphQL, depending on permissions.
GraphQL subscriptions
planned 2.x
Support for subscribing to real-time data
modification events, depending on permissions.
Tooling
Command-line tool
complete
The command-line tool can be used to export data
as SurrealQL, import data as SurrealQL, and
start a SurrealDB instance or cluster.
user@localhost % surreal .d8888b. 888 8888888b. 888888b. d88P Y88b 888 888 'Y88b 888 '88b Y88b. 888 888 888 888 .88P 'Y888b. 888 888 888d888 888d888 .d88b. 8888b. 888 888 888 8888888K. 'Y88b. 888 888 888P' 888P' d8P Y8b '88b 888 888 888 888 'Y88b '888 888 888 888 888 88888888 .d888888 888 888 888 888 888 Y88b d88P Y88b 888 888 888 Y8b. 888 888 888 888 .d88P 888 d88P 'Y8888P' 'Y88888 888 888 'Y8888 'Y888888 888 8888888P' 8888888P' To get started using SurrealDB, and for guides on connecting to and building applications on top of SurrealDB, check out the SurrealDB documentation (/docs/). If you have questions or ideas, join the SurrealDB community (/community). If you find a bug, submit an issue on GitHub (https://github.com/surrealdb/surrealdb/issues). We would love it if you could star the repository (https://github.com/surrealdb/surrealdb). ---------- Usage: surreal <COMMAND> Commands: start Start the database server backup Backup data to or from an existing database import Import a SurrealQL script into an existing database export Export an existing database as a SurrealQL script version Output the command-line tool and remote server version information upgrade Upgrade to the latest stable version sql Start an SQL REPL in your terminal with pipe support is-ready Check if the SurrealDB server is ready to accept connections [aliases: isready] validate Validate SurrealQL query files help Print this message or the help of the given subcommand(s) Options: -h, --help Print help
SQL export
complete
Export all data as SurrealQL from a SurrealDB
database for backup purposes. This includes
authentication scopes, tables, fields, events,
indexes, and data.
SQL import
complete
Import SurrealQL into a SurrealDB database in order
to restore from a backup. This includes
authentication scopes, tables, fields, events,
indexes, and data.
Incremental backups
future
Export all data from SurrealDB as raw binary data.
This will also support incremental binary backups
for efficient backing up of SurrealDB clusters.
Docker container
complete
In addition to binary releases, SurrealDB is
packaged as a Docker container for easy setup
and configuration. All configuration is done
with the command-line options. The Docker
container can be used to start a SurrealDB
instance or cluster, or to import and export
data.
docker run --rm --pull always -p 8000:8000 surrealdb/surrealdb:latest start
IDE language support
complete
Official SurrealQL language highlighting packages
for Visual Studio Code using TextMate grammar
definitions.
Language Server Protocol
future
Support for the Language Server Protocol will help
with code and query completion, and error
highlighting for SurrealQL.
User Interface
complete
An easy-to-use interface with support for
table-based views, SurrealQL querying, embedded
object editing, and graph visualisation. The
interface will be embedded within every
SurrealDB executable.
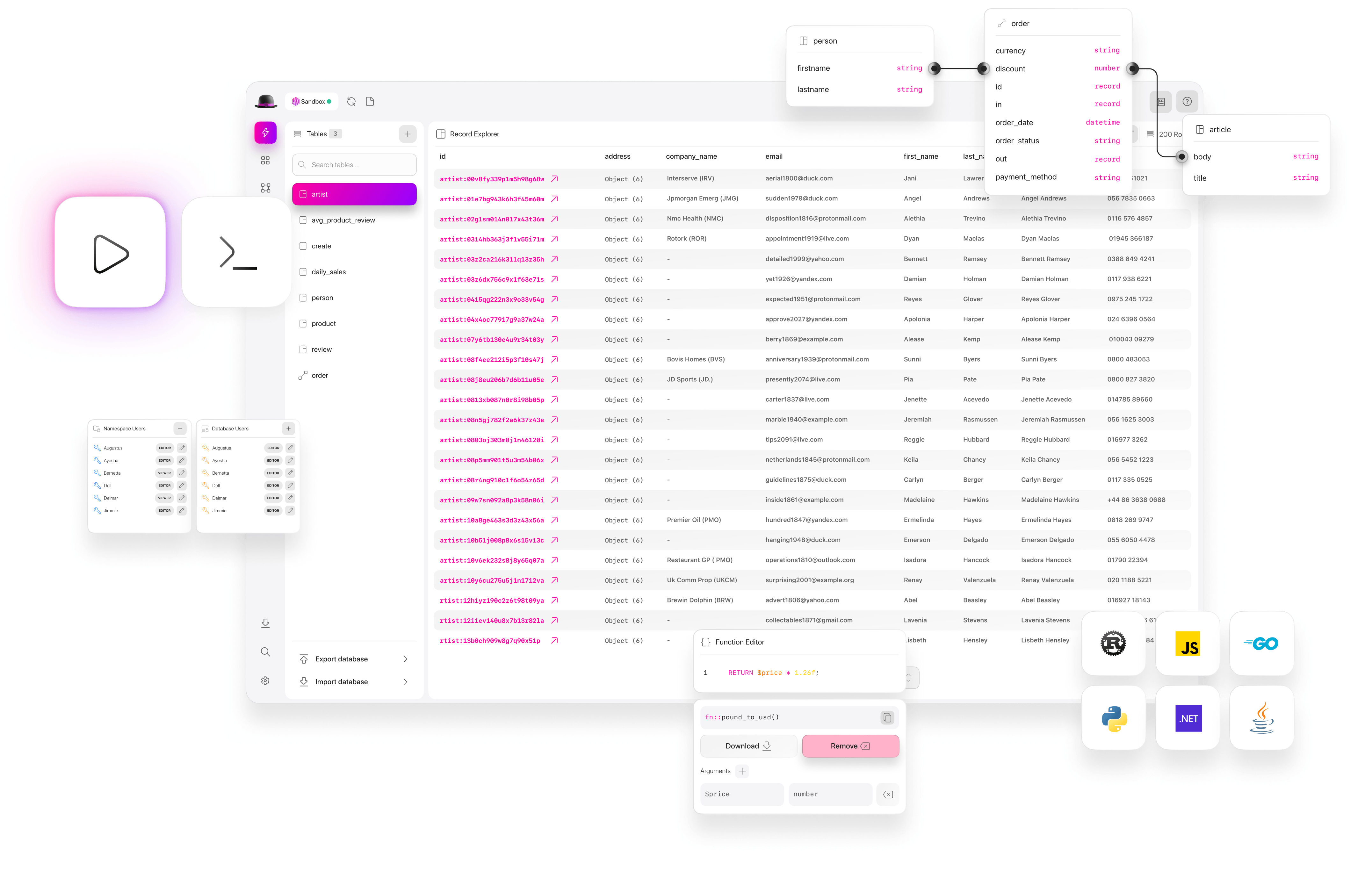
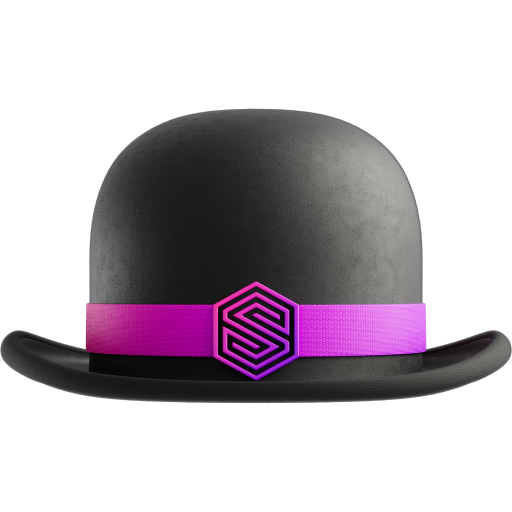
Web app
complete
The interface is available as a web app.
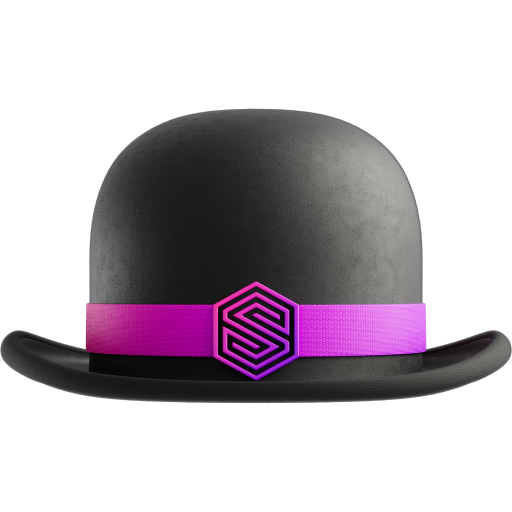
macOS
complete
The interface is available as a desktop application
for macOS powered by Tauri.
SDKs
Server-side SDKs
A native async-friendly SDK for Rust with
bi-directional, binary communication over WebSocket
or HTTP, and support for SurrealDB embedded
in-memory and on-disk.
A native SDK for JavaScript with bi-directional,
binary communication over WebSockets or HTTP, and
support for SurrealDB embedded in-memory and
on-disk.
Full support for TypeScript definitions from within
the JavaScript SDK, for working with strongly-typed
data with embedded and remote databases.
A native Node.js plugin for use with the JavaScript
SDK, enabling support for SurrealDB embedded
in-memory or on-disk using SurrealKV.
A native Deno plugin for use with the JavaScript
SDK, enabling support for SurrealDB embedded
in-memory or on-disk using SurrealKV.
An SDK for sync or async Python runtimes, with
binary communication over WebSocket or HTTP, and
support for SurrealDB embedded in-memory and
on-disk.
An SDK for Java with binary communication over
WebSocket or HTTP, and support for SurrealDB
embedded in-memory and on-disk.
An SDK for Golang with binary communication over
WebSocket or HTTP, and support for SurrealDB
embedded in-memory and on-disk.
A native SDK for .NET with bi-directional
communication over WebSockets or HTTP.
A native SDK for PHP with bi-directional, binary
communication over WebSockets or HTTP.
An SDK for C with binary communication over
WebSocket or HTTP, and support for SurrealDB
embedded in-memory and on-disk.
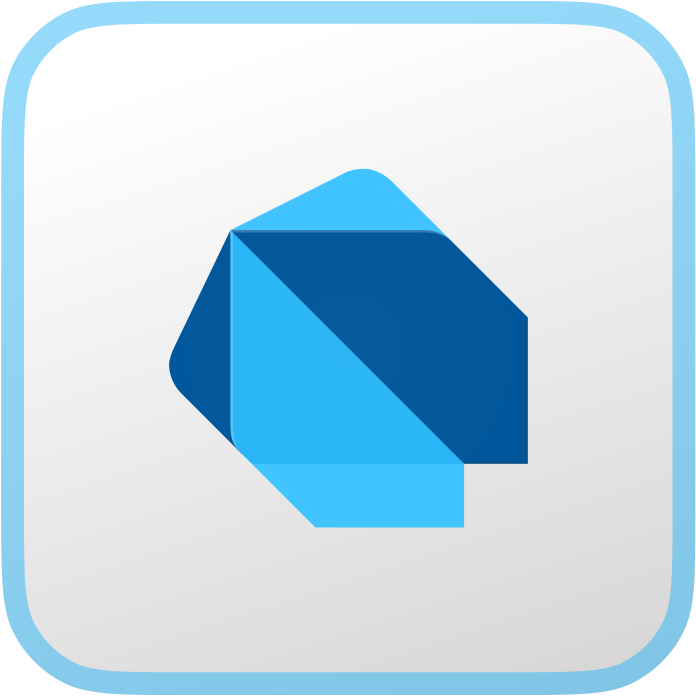
Dart
future
An SDK for Dart with binary communication over
WebSocket or HTTP, and support for SurrealDB
embedded in-memory and on-disk.
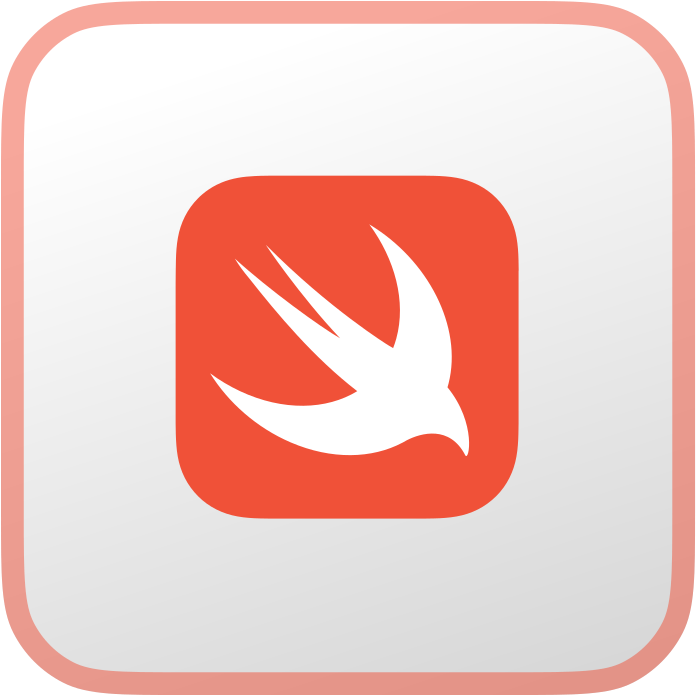
Swift
future
An SDK for Swift with binary communication over
WebSocket or HTTP.
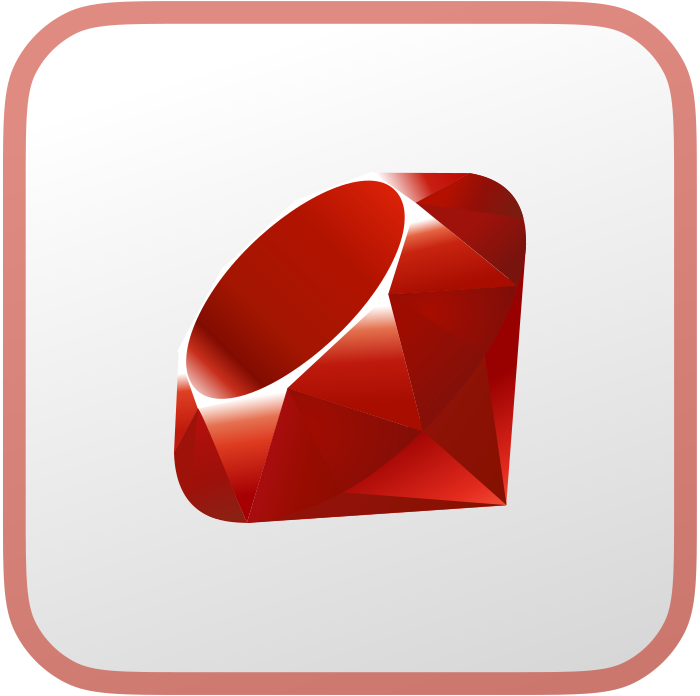
Ruby
future
An SDK for Ruby with binary communication over
WebSocket or HTTP.
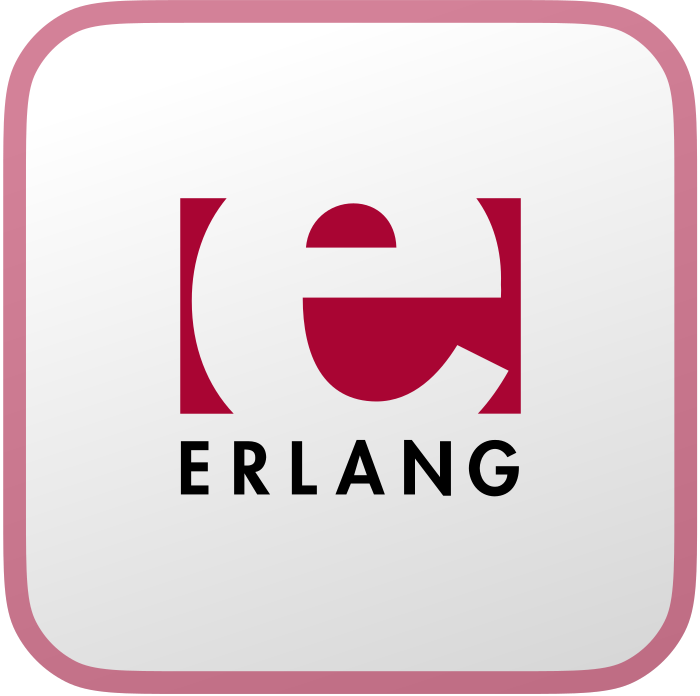
Erlang
future
An SDK for Erlang with bi-directional communication
over WebSockets.
Client-side SDKs
A native SDK for JavaScript with bi-directional,
binary communication over WebSockets or HTTP, and
support for SurrealDB embedded in-memory and
on-disk.
Full support for TypeScript definitions from within
the JavaScript SDK, for working with strongly-typed
data with embedded and remote databases.
A WebAssembly plugin for use with the JavaScript SDK
in the browser, enabling support for SurrealDB
embedded in-memory or persisted in IndexedDB.
A real-time, live-updating SDK for Ember.js, with
authentication, model definition, embedded types,
caching, and remote fetching.
Support for React.js using the native JavaScript
SDK, within TanStack Query, with support for data
caching and syncing, and authentication.
Support for Next.js using the native JavaScript SDK,
within TanStack Query, with support for data caching
and syncing, and authentication.
Support for Vue.js using the native JavaScript SDK,
within TanStack Query, with support for data caching
and syncing, and authentication.
Support for Angular using the native JavaScript SDK,
within TanStack Query, with support for data caching
and syncing, and authentication.
Support for Solid.js using the native JavaScript
SDK, within TanStack Query, with support for data
caching and syncing, and authentication.
Support for Svelte using the native JavaScript SDK,
within TanStack Query, with support for data caching
and syncing, and authentication.
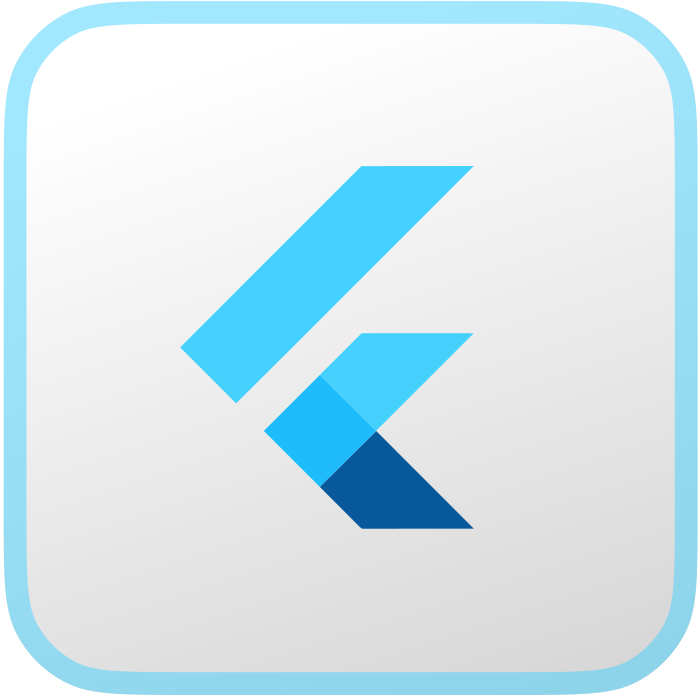
Flutter
future
An SDK for Flutter with bi-directional communication
over WebSockets.